Introduction
Resize the image and get the resized image in various formats such as Base64, Blob, image elements and so on.
Installation
Install with npm:
npm install --save js-scissor
Usage
See how to use js-scissor in the browser.
Resize width.
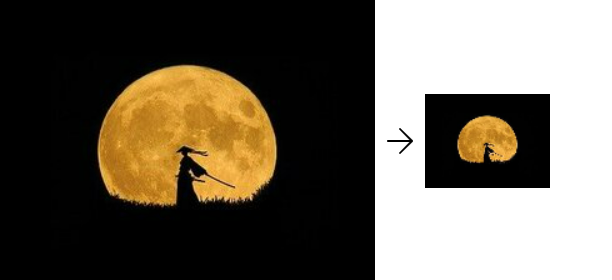
import Scissor from 'js-scissor';
// HTML: <img id="src1" src="img/sample1.jpg">
// <img id="dest1">
let src = document.querySelector('#src1');
let dest = document.querySelector('#dest1');
dest.src = (await new Scissor(src).resize(100)).toBase64();
Resize height.
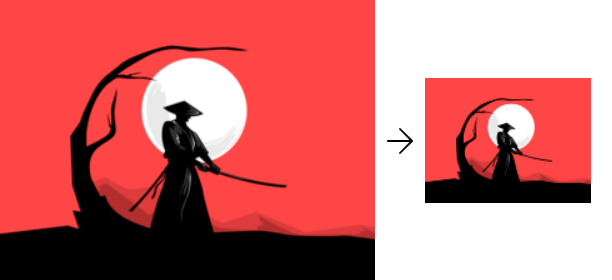
import Scissor from 'js-scissor';
// HTML: <img id="src2" src="img/sample2.png">
// <img id="dest2">
let src = document.querySelector('#src2');
let dest = document.querySelector('#dest2');
dest.src = (await new Scissor(src).resize(null, 100)).toBase64();
Resize by specifying width and height (cover)
Resize so that the center is cut off while maintaining the aspect ratio.
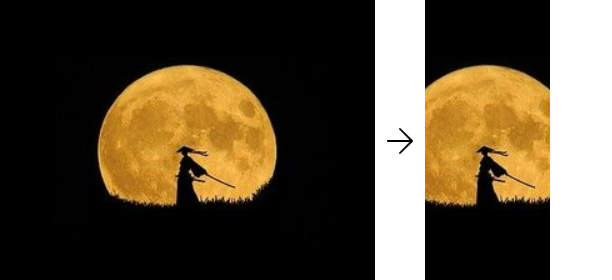
import Scissor from 'js-scissor';
// HTML: <img id="src3" src="img/sample1.jpg">
// <img id="dest3">
let src = document.querySelector('#src3');
let dest = document.querySelector('#dest3');
dest.src = (await new Scissor(src).resize(100, 225, {fit: 'cover'})).toBase64();
Resize by specifying width and height (contain)
Resize to fit the specified size while maintaining the aspect ratio.Blank areas are filled with the background color (default is black).
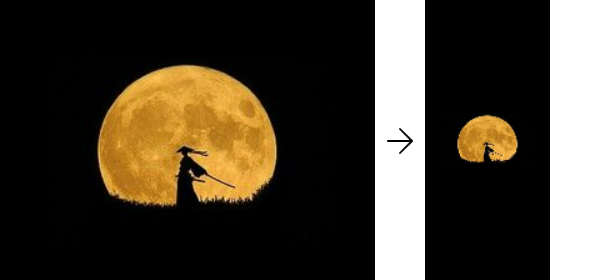
import Scissor from 'js-scissor';
// HTML: <img id="src4" src="img/sample1.jpg">
// <img id="dest4">
let src = document.querySelector('#src4');
let dest = document.querySelector('#dest4');
dest.src = (await new Scissor(src).resize(100, 225, {fit: 'contain'})).toBase64();
Resize by specifying width and height (fill)
Resize to the specified size without keeping the aspect ratio.
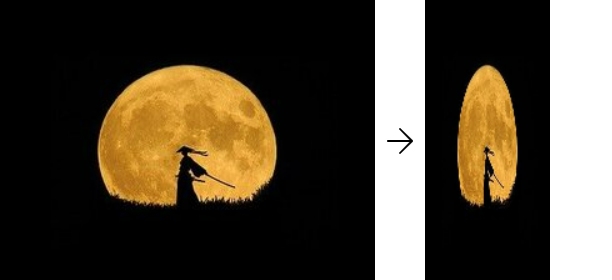
import Scissor from 'js-scissor';
// HTML: <img id="src5" src="img/sample1.jpg">
// <img id="dest5">
let src = document.querySelector('#src5');
let dest = document.querySelector('#dest5');
dest.src = (await new Scissor(src).resize(100, 225)).toBase64();
Resize the image read from the URL.
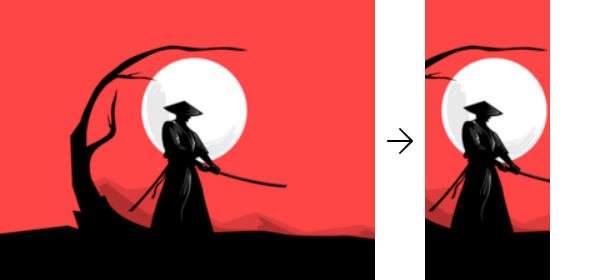
import Scissor from 'js-scissor';
// HTML: <img src="img/sample2.png">
// <img id="dest6">
let dest = document.querySelector('#dest6');
dest.src = (await new Scissor('img/sample2.png').resize(100, 225, {fit: 'cover'})).toBase64();
Class Scissor
This is a class that receives an image element, canvas element, and image URL in the constructor and outputs the edited result of the received image data.
Methods
Method | Description |
---|---|
public constructor(target: HTMLImageElement|HTMLCanvasElement|string) |
Constructs a Scissor object. Params:
Example:
|
public async resize( width: number|null, height?: number|null, opts?: { fit?: 'fill'|'cover'|'contain', background?: string, format?: 'image/webp'|'image/png'|'image/jpeg' } ): Promise<Output> |
Resizes the image and returns an "Output" object that can retrieve the resized image data in various formats. Params:
Return: {Promise<Output>} Returns an "output" object that can retrieve resized image data in various formats. Example:
|
Class Output
A class that converts resized images into various formats.
Methods
Method | Description |
---|---|
public constructor(cvs: HTMLCanvasElement, format: 'image/webp'|'image/png'|'image/jpeg') |
Constructs a Output object. Params:
|
public toBase64(): string |
Returns the resized image in base64 format. Return: {string} Base64 data of the image after resizing, e.g. data:image/png;base64, iVB... Example:
|
public toImage(): HTMLImageElement |
Returns the resized image as an image element. Return: {HTMLImageElement} The image element of the resized image. Example:
|
public toBlob(): Blob |
Returns the blob data for the resized image. Return: {Blob} Blob data for resized images. Example:
|